class 是 ES6 的新特性,可以用来定义一个类,实际上,class 只是一种语法糖,它是构造函数的另一种写法。(什么是语法糖?是一种为避免编码出错和提高效率编码而生的语法层面的优雅解决方案,简单说就是,一种便携写法。)
1 2 3
| class Person {} typeof Person Person.prototype.constructor === Person
|
上面代码表明,类的数据类型就是函数,类本身就指向构造函数。
基础介绍
使用
使用的时候,也是直接对类使用new命令,跟构造函数的用法完全一致。
1 2 3 4 5 6 7
| class Bar { doStuff() { console.log('stuff'); } } const b = new Bar(); b.doStuff()
|
构造函数的prototype
属性,在 ES6 的“类”上面继续存在。事实上,类的所有方法都定义在类的prototype
属性上面。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class Point { constructor() { toString() { toValue() { }
Point.prototype = { constructor() {}, toString() {}, toValue() {}, };
|
在类的实例上面调用方法,其实就是调用原型上的方法:
1 2 3
| class B {} let b = new B(); b.constructor === B.prototype.constructor
|
通过Object.assign
方法可以很方便地一次向类添加多个方法:
1 2 3 4 5 6 7 8
| class Point { constructor(){ } Object.assign(Point.prototype, { toString(){}, toValue(){} });
|
prototype
对象的constructor
属性,直接指向“类”的本身:Point.prototype.constructor === Point // true
而且,类的内部所有定义的方法,都是不可枚举的(non-enumerable):
1 2 3 4 5 6 7 8
| class Point { constructor(x, y) { toString() { } Object.keys(Point.prototype) Object.getOwnPropertyNames(Point.prototype)
|
注意:上面代码中,toString
方法是Point类内部定义的方法,它是不可枚举的。这一点与 ES5 (构造函数)的行为不一致。
1 2 3 4 5 6
| const Point = function (x, y) { Point.prototype.toString = function() { Object.keys(Point.prototype) Object.getOwnPropertyNames(Point.prototype)
|
constructor
constructor
方法是类的默认方法,通过new
命令生成对象实例时,自动调用该方法。
一个类必须有constructor
方法,如果没有显式定义,一个空的constructor
方法会被默认添加。
1 2 3 4 5
| class Point { }
constructor() {} }
|
上面代码中,定义了一个空的类Point,JavaScript 引擎会自动为它添加一个空的constructor
方法。constructor
方法默认返回实例对象(即this
),完全可以指定返回另外一个对象。
1 2 3 4 5 6
| class Foo { constructor() { return Object.create(null); } } new Foo() instanceof Foo
|
上面代码中,constructor
函数返回一个全新的对象,结果导致实例对象不是Foo类的实例。
类必须使用new
调用,否则会报错。这是它跟普通构造函数的一个主要区别,后者不用new
也可以执行。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class Foo { constructor() { return Object.create(null); } } console.log(Foo());
function Foo(){ return Object.create(null); } console.log(Foo());
|
类的实例
生成类的实例的写法,与 ES5 构造函数完全一样,也是使用new
命令。如果忘记加上new
,像函数那样调用Class会报错。
与 ES5构造函数 一样,实例的属性除非显式定义在其本身(即定义在this对象上),否则都是定义在原型上(即定义在class上) 。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Point { constructor(x, y) { this.x = x; this.y = y; } toString() { return '(' + this.x + ', ' + this.y + ')'; } } let point = new Point(2, 3); point.toString() point.hasOwnProperty('x') point.hasOwnProperty('y') point.hasOwnProperty('toString') point.__proto__.hasOwnProperty('toString')
|
上面代码中,x
和y
都是实例对象point
自身的属性(因为定义在this
变量上),所以hasOwnProperty
方法返回true
,而toString
是原型对象的属性(因为定义在Point
类上),所以hasOwnProperty
方法返回false
。
与 ES5 构造函数一样,类的所有实例共享一个原型对象。
1 2 3 4
| let p1 = new Point(2,3); let p2 = new Point(3,2); p1.__proto__ === p2.__proto__
|
注意: __proto__
并不是语言本身的特性,这是各大厂商具体实现时添加的私有属性,虽然目前很多现代浏览器的 JS 引擎中都提供了这个私有属性,但依旧不建议在生产中使用该属性,避免对环境产生依赖。生产环境中,可以使用 Object.getPrototypeOf
方法来获取实例对象的(隐式)原型,然后再来为原型添加方法/属性。
可以通过实例的 __proto__
属性为“类”添加方法:
1 2 3 4 5 6 7
| let p1 = new Point(2,3); let p2 = new Point(3,2); p1.__proto__.printName = function () { return 'Oops' };
p1.printName() let p3 = new Point(4,2); p3.printName()
|
上面代码在p1
的原型上添加了一个printName
方法,由于p1
的原型就是p2
的原型,因此p2
也可以调用这个方法。而且,此后新建的实例p3
也可以调用这个方法。这意味着,使用实例的__proto__
属性改写原型,必须相当谨慎,不推荐使用,因为这会改变“类”的原始定义,影响到所有实例。
取值函数(getter)和存值函数(setter)
与 ES5构造函数 一样,在“类”的内部可以使用get
和set
关键字,对某个属性设置存值函数和取值函数,拦截该属性的存取行为。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class MyClass { constructor() { } get prop() { return 'getter'; } set prop(value) { console.log('setter: '+value); } } let inst = new MyClass(); inst.prop = 123; console.log(inst.prop);
|
存值函数和取值函数是设置在属性的 Descriptor 对象上的。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class CustomHTMLElement { constructor(element) { this.element = element; } get html() { return this.element.innerHTML; } set html(value) { this.element.innerHTML = value; } } let descriptor = Object.getOwnPropertyDescriptor( CustomHTMLElement.prototype, "html" ); "get" in descriptor "set" in descriptor console.log(descriptor);
|
属性和方法
定义于 constructor
内的属性和方法,即定义在 this
上,属于实例属性和方法,否则属于原型属性和方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class Person { constructor (name) { this.name = name }
say () { console.log('hello') } }
let jon = new Person()
jon.hasOwnProperty('name') jon.hasOwnProperty('say')
|
属性表达式
类的属性名,可以采用表达式。
1 2 3 4 5 6 7 8 9 10
| let methodName = 'say' class Person { constructor (name) { this.name = name }
[methodName] () { console.log('hello') } }
|
Class表达式
与函数一样,类也可以使用表达式的形式定义。
1 2 3 4 5
| const MyClass = class Me { getClassName() { return Me.name; } };
|
需要注意的是,这个类的名字是Me
,但是Me
只在 Class
的内部可用,指代当前类。
在 Class
外部,这个类只能用MyClass
引用。
1 2 3 4
| let inst = new MyClass(); inst.getClassName() Me.name
|
Me只在 Class 内部有定义。如果类的内部没用到的话,可以省略Me
,也就是可以写成下面的形式:(简写)const MyClass = class { /* ... */ };
采用 Class 表达式,可以写出立即执行的 Class:
1 2 3 4 5 6 7 8 9
| let person = new class { constructor(name) { this.name = name; } sayName() { console.log(this.name); } }('张三'); person.sayName();
|
person
是一个立即执行的类的实例。
注意点
严格模式
类和模块的内部,默认就是严格模式,所以不需要使用use strict
指定运行模式。
不存在变量提升
类不存在变量提升(hoist) ,这一点与 ES5 构造函数完全不同。
类必须先定义再使用,这种规定的原因与下文要提到的继承有关,必须保证子类在父类之后定义。
1 2 3 4 5
| { let Foo = class {}; class Bar extends Foo { } }
|
上面的代码不会报错,因为Bar继承Foo的时候,Foo已经有定义了。但是,如果存在class的提升,上面代码就会报错,因为class会被提升到代码头部,而let
命令是不提升的,所以导致Bar继承Foo的时候,Foo还没有定义。
name
属性
由于本质上,ES6 的类只是 ES5 的构造函数的一层包装,所以函数的许多特性都被Class继承,包括name
属性。
1 2 3
| class Point {} Point.name
|
Generator方法
如果给类的某个方法之前加上星号(*
),就表示该方法是一个 Generator 函数。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class Foo { constructor(...args) { this.args = args; } * [Symbol.iterator]() { for (let arg of this.args) { yield arg; } } } for (let x of new Foo('hello', 'world')) { console.log(x); }
|
Symbol.iterator
方法返回一个Foo类的默认遍历器,for...of
循环会自动调用这个遍历器。
this
的指向
类的方法内部如果含有this
,它默认指向类的实例。但是,必须非常小心,一旦单独使用该方法,很可能报错。
1 2 3 4 5 6 7 8 9 10 11
| class Logger { printName(name = 'there') { this.print(`Hello ${name}`); } print(text) { console.log(text); } } const logger = new Logger(); const { printName } = logger; printName();
|
上面代码中,printName
方法中的this
,默认指向Logger类的实例。
但是,如果将这个方法提取出来单独使用,this
会指向该方法运行时所在的环境(由于 class 内部是严格模式,所以 this
实际指向的是undefined
),从而导致找不到print
方法而报错。
解决办法:
- 在构造方法中绑定
this
1 2 3 4 5 6
| class Logger { constructor() { this.printName = this.printName.bind(this); } }
|
- 使用箭头函数:下面代码中,箭头函数位于构造函数内部,它的定义生效的时候,是在构造函数执行的时候。这时,箭头函数所在的运行环境,肯定是实例对象,所以
this
会总是指向实例对象。
1 2 3 4 5 6 7 8 9 10 11
| class Obj { constructor() { this.getThis = () => this; } } const myObj = new Obj(); console.log(myObj.getThis() === myObj); const { getThis } = myObj console.log(getThis());
|
- 使用Proxy,获取方法的时候,自动绑定
this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| class Logger { printName(name = 'there') { this.print(`Hello ${name}`); } print(text) { console.log(text); } } function selfish (target) { const cache = new WeakMap(); const handler = { get (target, key) { const value = Reflect.get(target, key); if (typeof value !== 'function') { return value; } if (!cache.has(value)) { cache.set(value, value.bind(target)); } return cache.get(value); } }; const proxy = new Proxy(target, handler); return proxy; } const logger = selfish(new Logger()); const {printName} = logger printName('proxy')
|
静态方法
类相当于实例的原型,所有在类中定义的方法,都会被实例继承。如果在一个方法前,加上static
关键字,就表示该方法不会被实例继承,而是直接通过类来调用,这就称为“静态方法” 。如果静态方法包含this
关键字, 其中的this
指向类本身。
1 2 3 4 5 6 7 8 9 10 11 12
| class Foo { static bar() { this.baz(); } static baz() { console.log('hello'); } baz() { console.log('world'); } } Foo.bar()
|
静态方法可以与非静态方法重名。父类的静态方法,可以被子类继承。
1 2 3 4 5 6 7 8
| class Foo { static classMethod() { return 'hello'; } } class Bar extends Foo { } Bar.classMethod()
|
静态方法也是可以从super
对象上调用的:
1 2 3 4 5 6 7 8 9 10 11
| class Foo { static classMethod() { return 'hello'; } } class Bar extends Foo { static classMethod() { return super.classMethod() + ', too'; } } Bar.classMethod()
|
实例属性新写法
实例属性除了定义在constructor()
方法里面的this
上面,也可以定义在类的最顶层,其他都不变。
1 2 3 4 5 6 7 8 9 10 11 12
| class IncreasingCounter { constructor() { this._count = 0; } get value() { console.log('Getting the current value!'); return this._count; } increment() { this._count++; } }
|
1 2 3 4 5 6 7 8 9 10 11 12
| class IncreasingCounter { _count = 0; bar = 'hello'; baz = 'world'; get value() { console.log('Getting the current value!'); return this._count; } increment() { this._count++; } }
|
这种新写法的好处是,所有实例对象自身的属性都定义在类的头部,看上去比较整齐,一眼就能看出这个类有哪些实例属性。
静态属性
静态属性指的是 Class 本身的属性,即Class.propName
,而不是定义在实例对象(this
)上的属性。
1 2 3 4
| class Foo { } Foo.prop = 1; Foo.prop
|
1 2 3 4 5 6 7 8
| class MyClass { static myStaticProp = 42; constructor() { console.log(MyClass.myStaticProp); } }
console.log(MyClass.myStaticProp);
|
私有方法和私有属性
私有方法和私有属性,是只能在类的内部访问的方法和属性, 外部不能访问。
老式解决方案
- 在命名上加以区别:使用
_
表示私有的属性或方法
这种命名是不保险的,在类的外部,还是可以调用到这个方法,这样写只是让开发人员看到是一个私有属性或方法,不要在外部调用。
1 2 3 4 5 6 7 8 9
| class Widget { this._bar(baz); } return this.snaf = baz; } }
|
将私有方法移出模块
下面代码中,foo
是公开方法,内部调用了bar.call(this, baz)
。这使得bar
实际上成为了当前模块的私有方法。但是和上面一样,外面依然可以进行调用。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| class Widget { foo (baz) { console.log(bar.call(this, baz)); } } function bar(baz) { return this.snaf = baz; } const widget = new Widget(); widget.foo('baz'); console.log(widget.snaf);
const obj = {snaf:'snaf'} console.log(bar.call(obj, 'baz')); console.log(obj.snaf);
|
- 利用Symbol值的唯一性,将私有方法的名字命名为一个Symbol值
上面代码中,bar
和snaf
都是Symbol值,一般情况下无法获取到它们,因此达到了私有方法和私有属性的效果。但是也不是绝对不行,Reflect.ownKeys()
依然可以拿到它们。
1 2 3 4 5 6 7 8 9 10 11 12 13
| const bar = Symbol('bar'); const snaf = Symbol('snaf'); export default class myClass{ foo(baz) { this[bar](baz); } [bar](baz) { return this[snaf] = baz; } };
|
1 2
| const inst = new myClass(); Reflect.ownKeys(myClass.prototype)
|
新式解决方案
在属性名或方法名之前,使用 #
表示私有属性或方法。这种是严格的私有,在外部获取或调用就会报错。
1 2 3 4 5 6 7 8 9 10 11 12 13
| class IncreasingCounter { #count = 0; get value() { console.log('Getting the current value!'); return this.#count; } increment() { this.#count++; } } const counter = new IncreasingCounter(); counter.#count counter.#count = 42
|
私有属性不限于从this
引用,只要是在类的内部,实例也可以引用私有属性。
1 2 3 4 5 6 7
| class Foo { #privateValue = 42; static getPrivateValue(foo) { return foo.#privateValue; } } Foo.getPrivateValue(new Foo());
|
私有属性和私有方法前面,也可以加上static
关键字,表示这是一个静态的私有属性或私有方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| class FakeMath { static PI = 22 / 7; static #totallyRandomNumber = 4; static #computeRandomNumber() { return FakeMath.#totallyRandomNumber; } static random() { console.log('I heard you like random numbers…') return FakeMath.#computeRandomNumber(); } } FakeMath.PI FakeMath.random()
FakeMath.#totallyRandomNumber FakeMath.#computeRandomNumber()
|
上面代码中,#totallyRandomNumber
是私有属性,#computeRandomNumber()
是私有方法,只能在FakeMath这个类的内部调用,外部调用就会报错。
new.target
属性
ES6 为new命令引入了一个new.target
属性,该属性一般用在构造函数之中,返回new
命令作用于的那个构造函数。如果构造函数不是通过new
命令或Reflect.construct()
调用的, new.target
会返回undefined
,因此这个属性可以用来确定构造函数是怎么调用的。
注意:在函数外部,使用new.target
会报错。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| function Person(name) { if (new.target !== undefined) { this.name = name; } else { throw new Error('必须使用 new 命令生成实例'); } }
function Person(name) { if (new.target === Person) { this.name = name; console.log('new.target:', new.target); } else { console.log(new.target); console.log('必须使用 new 命令生成实例'); throw new Error('必须使用 new 命令生成实例'); } } let person = new Person('张三'); let notAPerson = Person.call(person, '张三');
|
Class 内部调用new.target
,返回当前 Class。
1 2 3 4 5 6 7 8
| class Rectangle { constructor(length, width) { console.log(new.target === Rectangle); this.length = length; this.width = width; } } const obj = new Rectangle(3, 4);
|
子类继承父类时, new.target
会返回子类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class Rectangle { constructor(length, width) { console.log(new.target); } } class Square extends Rectangle { constructor(length, width) { super(length, width); } } let obj = new Square(3);
|
利用这个特点,可以写出 不能独立使用、必须继承后才能使用的类。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class Shape { constructor() { if (new.target === Shape) { throw new Error('本类不能实例化'); } } } class Rectangle extends Shape { constructor(length, width) { super(); } } let x = new Shape();
let y = new Rectangle(3, 4); console.log(y); console.log(y instanceof Shape);
|
上面代码中,Shape类不能被实例化,只能用于继承。
继承
Class 可以通过extends
关键字实现继承,这比 ES5 的通过修改原型链实现继承,要清晰和方便很多。
1 2
| class Point {} class ColorPoint extends Point {}
|
Object.getPrototypeOf()
与Object.setPrototypeOf()
Object.setPrototypeOf()
也可以用来实现继承,而Object.getPrototypeOf()
可以用来从子类上获取父类。
1 2 3 4
| class Point {} class ColorPoint extends Point{}
console.log(Object.getPrototypeOf(ColorPoint));
|
1 2 3 4
| class Point {} class ColorPoint{} Object.setPrototypeOf(ColorPoint, Point); console.log(Object.getPrototypeOf(ColorPoint));
|
上面两种继承方式等价。
super
关键字
super
是一个函数,子类中的super
就是父类中constructor
构造器的一个引用。
super
这个关键字,既可以当作函数使用,也可以当作对象使用。在这两种情况下,它的用法完全不同。
super
作为函数调用
super
作为函数调用时,代表父类的构造函数。ES6 要求,子类的构造函数必须执行一次super
函数。
子类必须在constructor
方法中调用super
方法,否则新建实例时会报错。这是因为子类自己的this
对象,必须先通过父类的构造函数完成塑造,得到与父类同样的实例属性和方法,然后再对其进行加工,加上子类自己的实例属性和方法。如果不调用super
方法,子类就得不到this
对象。
注意:super
虽然代表了父类的构造函数,但是返回的是子类的实例,即super
内部的this
指的是子类的实例,因此super()
相当于父类.prototype.constructor.call(this)
。
- ES5 的继承,实质是先创造子类的实例对象
this
,然后再将父类的方法添加到this上面(Parent.apply(this))。
- ES6 的继承机制完全不同,实质是先将父类实例对象的属性和方法,加到
this
上面(所以必须先调用super
方法),然后再用子类的构造函数修改this
。
1 2 3 4 5 6
| class Point { } class ColorPoint extends Point { constructor() { } } let cp = new ColorPoint();
|
如果子类没有定义constructor
方法,这个方法会被默认添加,代码如下。也就是说,不管有没有显式定义,任何一个子类都有constructor
方法:
1 2 3 4 5 6 7 8
| class ColorPoint extends Point { }
class ColorPoint extends Point { constructor(...args) { super(...args); } }
|
在子类的构造函数中,只有调用super
之后,才可以使用this
关键字,否则会报错。这是因为子类实例的构建,基于父类实例,只有super
方法才能调用父类实例:
1 2 3 4 5 6 7 8 9 10 11 12
| class Point { constructor(x, y) { this.x = x; this.y = y; } } class ColorPoint extends Point { constructor(x, y, color) { this.color = color; super(x, y); this.color = color; }
|
实例对象同时是父类与子类的实例,这与 ES5 的行为完全一致:
1 2 3
| let cp = new ColorPoint(25, 8, 'green'); cp instanceof ColorPoint cp instanceof Point
|
父类的静态方法,也会被子类继承:
1 2 3 4 5 6 7 8
| class A { static hello() { console.log('hello world'); } } class B extends A { } B.hello()
|
super()
内部的this
指向的是子类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| class A { constructor() { console.log(new.target.name); } } class B extends A { constructor() { super(); } } new A() new B()
|
作为函数时, super()
只能用在子类的构造函数之中,用在其他地方就会报错:
1 2 3 4 5 6
| class A {} class B extends A { m() { super(); } }
|
super
作为对象
super
作为对象时:
- 在普通方法中,指向 父类的原型对象
- 在静态方法中,指向 父类。
1 2 3 4 5 6 7 8 9 10 11 12
| class A { p() { return 2; } } class B extends A { constructor() { super(); console.log(super.p()); } } let b = new B();
|
上面代码中,子类B当中的super.p()
,就是将super
当作一个对象使用。这时,super
在普通方法之中,指向A.prototype
,所以super.p()
就相当于A.prototype.p()
。
由于super
指向父类的原型对象,所以定义在父类实例上的方法或属性,是无法通过super
调用的:
1 2 3 4 5 6 7 8 9 10 11 12
| class A { constructor() { this.p = 2; } } class B extends A { get m() { return super.p; } } let b = new B(); b.m
|
如果属性定义在父类的原型对象上, super
就可以取到:
1 2 3 4 5 6 7 8 9
| class A {} A.prototype.x = 2; class B extends A { constructor() { super(); console.log(super.x) } } let b = new B();
|
ES6 规定,在子类普通方法中通过super
调用父类的方法时,方法内部的this
指向当前的子类实例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class A { constructor() { this.x = 1; } print() { console.log(this.x); } } class B extends A { constructor() { super(); this.x = 2; } m() { super.print(); } } let b = new B(); b.m()
|
上面代码中,super.print()
虽然调用的是A.prototype.print()
,但是A.prototype.print()
内部的this
指向子类B的实例,导致输出的是2,而不是1。也就是说,实际上执行的是super.print.call(this)
。
由于this
指向子类实例,所以如果通过super
对某个属性赋值,这时super
就是this
,赋值的属性会变成子类实例的属性:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class A { constructor() { this.x = 1; } } class B extends A { constructor() { super(); this.x = 2; super.x = 3; console.log(super.x); console.log(this.x); } } let b = new B();
|
上面代码中,super.x
赋值为3,这时等同于对this.x
赋值为3。而当读取super.x
的时候,读的是A.prototype.x
,因为A里面的x
是实例上的,不是原型对象上的,所以返回undefined
。
如果super
作为对象,用在静态方法之中,这时super
将指向父类,而不是父类的原型对象:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class Parent { static myMethod(msg) { console.log('static', msg); } myMethod(msg) { console.log('instance', msg); } } class Child extends Parent { static myMethod(msg) { super.myMethod(msg); } myMethod(msg) { super.myMethod(msg); } } Child.myMethod(1); const child = new Child(); child.myMethod(2);
|
在子类的静态方法中通过super
调用父类的方法时,方法内部的this
指向当前的子类 ,而不是子类的实例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class A { constructor() { this.x = 1; } static print() { console.log(this.x); } } class B extends A { constructor() { super(); this.x = 2; } static m() { super.print(); } } B.x = 3; B.m()
|
上面代码中,静态方法B.m
里面,super.print
指向父类的静态方法。这个方法里面的this
指向的是B,而不是B的实例。
注意:使用super
的时候,必须显式指定是作为函数、还是作为对象使用,否则会报错:
1 2 3 4 5 6 7
| class A {} class B extends A { constructor() { super(); console.log(super); } }
|
上面代码中,console.log(super)
当中的super
,无法看出是作为函数使用,还是作为对象使用,所以 JavaScript 引擎解析代码的时候就会报错。
这时,如果能清晰地表明super
的数据类型,就不会报错。
1 2 3 4 5 6 7 8 9 10
| class A {} class B extends A { constructor() { super(); console.log(super.valueOf() instanceof B); } } let b = new B();
|
由于对象总是继承其他对象的,所以可以在任意一个对象中,使用super
关键字:
1 2 3 4 5 6
| const obj = { toString() { return "MyObject: " + super.toString(); } }; obj.toString();
|
类的 prototype
属性和__proto__
属性
大多数浏览器的 ES5 实现之中,每一个对象都有__proto__
属性,指向对应的构造函数的prototype
属性。
Class 作为构造函数的语法糖,同时有prototype
属性和__proto__
属性,因此同时存在两条继承链。
- 子类的
__proto__
属性,表示构造函数的继承,总是指向父类。
- 子类
prototype
属性的 __proto__
属性,表示方法的继承,总是指向父类的prototype
属性。
1 2 3 4 5 6 7 8 9 10 11
| class A {} A.prototype.Hi = function (){ console.log('Hi'); } class B extends A {} console.log(B.__proto__); console.log(A.__proto__); console.log(B.prototype.__proto__); console.log(A.prototype); B.__proto__ === A B.prototype.__proto__ === A.prototype
|
上面代码中,子类B的__proto__
属性指向父类A,子类B的prototype
属性的__proto__
属性指向父类A的prototype
属性。
类的继承是按照下面的模式实现的:
1 2 3 4 5 6 7 8 9
| class A { } class B { }
Object.setPrototypeOf(B.prototype, A.prototype);
Object.setPrototypeOf(B, A); const b = new B();
|
1 2 3 4
| Object.setPrototypeOf = function (obj, proto) { obj.__proto__ = proto; return obj; }
|
1 2 3 4 5 6 7
| Object.setPrototypeOf(B.prototype, A.prototype);
B.prototype.__proto__ = A.prototype;
Object.setPrototypeOf(B, A);
B.__proto__ = A;
|
这两条继承链,可以这样理解:
- 作为一个对象,子类(B)的原型(
__proto__
属性)是父类(A) ;
- 作为一个构造函数,子类(B)的原型对象(
prototype
属性)是父类的原型对象( prototype
属性)的 实例。
1 2 3
| B.prototype = Object.create(A.prototype);
B.prototype.__proto__ = A.prototype;
|
extends
关键字后面可以跟多种类型的值:class B extends A {}
上面代码的A,只要是一个有prototype
属性的函数,就能被B继承。由于函数都有prototype属性( 除了Function.prototype
函数 ),因此A可以是任意函数:
- 子类继承Object类:这种情况下,A其实就是构造函数
Object
的复制,A的实例就是Object
的实例。
1 2 3
| class A extends Object {} A.__proto__ === Object A.prototype.__proto__ === Object.prototype
|
- 不存在任何继承:这种情况下,A作为一个基类(即不存在任何继承),就是一个普通函数,所以直接继承
Function.prototype
。但是,A调用后返回一个空对象(即Object
实例),所以A.prototype.__proto__
指向构造函数(Object
)的prototype
属性。
1 2 3
| class A {} A.__proto__ === Function.prototype A.prototype.__proto__ === Object.prototype
|
实例的 __proto__
属性
子类实例的__proto__
属性的__proto__
属性,指向父类实例的__proto__
属性。也就是说,子类的原型的原型,是父类的原型。
1 2 3 4 5
| class ColorPoint extends Point{} let p1 = new Point(2, 3); let p2 = new ColorPoint(2, 3, 'red'); p2.__proto__ === p1.__proto__ p2.__proto__.__proto__ === p1.__proto__
|
上面代码中,ColorPoint
继承了Point
,导致前者原型的原型是后者的原型。
通过子类实例的 __proto__.__proto__
属性,可以修改父类实例的行为:
1 2 3 4
| p2.__proto__.__proto__.printName = function () { console.log('Ha'); }; p1.printName()
|
上面代码在ColorPoint
的实例p2
上向Point
类添加方法,结果影响到了Point
的实例p1
。
原生构造函数的继承
原生构造函数是指语言内置的构造函数,通常用来生成数据结构。
Boolean()
Number()
String()
Array()
Date()
Function()
RegExp()
Error()
Object()
以前,这些原生构造函数是无法继承的:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| function MyArray() { Array.apply(this, arguments); } MyArray.prototype = Object.create(Array.prototype, { constructor: { value: MyArray, writable: true, configurable: true, enumerable: true } });
let colors = new MyArray(); colors[0] = "red"; colors.length colors.length = 0; colors[0]
|
上面代码定义了一个继承 Array 的MyArray类。但是,这个类的行为与Array完全不一致。
这是因为子类无法获得原生构造函数的内部属性,通过Array.apply()
或者分配给原型对象都不行。原生构造函数会忽略apply
方法传入的this
,也就是说,原生构造函数的this
无法绑定,导致拿不到内部属性。
ES5 是先新建子类的实例对象this
,再将父类的属性添加到子类上,由于父类的内部属性无法获取,导致无法继承原生的构造函数。
ES6 允许继承原生构造函数定义子类,因为 ES6 是先新建父类的实例对象this
,然后再用子类的构造函数修饰this
,使得父类的所有行为都可以继承。这意味着,ES6 可以自定义原生数据结构(比如Array、String等)的子类。
1 2 3 4 5 6 7 8 9 10
| class MyArray extends Array { constructor(...args) { super(...args); } } const arr = new MyArray(); arr[0] = 12; arr.length arr.length = 0; arr[0]
|
extends
关键字不仅可以用来继承类,还可以用来继承原生的构造函数。因此可以在原生数据结构的基础上,定义自己的数据结构:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| class VersionedArray extends Array { constructor() { super(); this.history = [[]]; } commit() { this.history.push(this.slice()); } revert() { this.splice(0, this.length, ...this.history.at(-1)); } } var x = new VersionedArray(); x.push(1); x.push(2); console.log(x);
x.commit(); console.log(x.history);
x.push(3); console.log(x)
x.revert(); console.log(x)
|
上面代码中,VersionedArray会通过commit
方法,将自己的当前状态生成一个版本快照,存入history
属性。revert
方法用来将数组重置为最新一次保存的版本。除此之外,VersionedArray依然是一个普通数组,所有原生的数组方法都可以在它上面调用。
1 2 3 4 5 6 7 8 9 10 11 12 13
| class myError extends Error { constructor(message){ super() this.message = message this.stack = (new Error()).stack this.name = this.constructor.name } }
const error = new myError('自定义错误') console.log(error.message); console.log(error.stack); console.log(error.name);
|
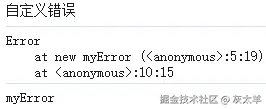
注意:继承Object的子类,有一个行为差异:
1 2 3 4 5 6 7
| class NewObj extends Object{ constructor(){ super(...arguments); } } const o = new NewObj({attr: true}); o.attr === true
|
上面代码中,NewObj
继承了Object
,但是无法通过super
方法向父类Object
传参。这是因为 ES6 改变了Object
构造函数的行为,一旦发现Object
方法不是通过new Object()
这种形式调用,ES6 规定Object
构造函数会忽略参数。
Mixin 模式的实现
Mixin 指的是多个对象合成一个新的对象,新对象具有各个组成成员的接口。
1 2 3 4 5 6 7 8
| const a = { a: 'a' }; const b = { b: 'b' }; const c = {...a, ...b};
|
下面是一个更完备的实现,将多个类的接口“混入”(mixin)另一个类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| function mix(...mixins) { class Mix { constructor() { for (let mixin of mixins) { copyProperties(this, new mixin()); } } } for (let mixin of mixins) { copyProperties(Mix, mixin); copyProperties(Mix.prototype, mixin.prototype); } return Mix; } function copyProperties(target, source) { for (let key of Reflect.ownKeys(source)) { if ( key !== 'constructor' && key !== 'prototype' && key !== 'name' ) { let desc = Object.getOwnPropertyDescriptor(source, key); Object.defineProperty(target, key, desc); } } }
|
上面代码的mix函数,可以将多个对象合成为一个类。使用的时候,只要继承这个类即可:
1 2 3
| class DistributedEdit extends mix(Loggable, Serializable) { }
|